What's new in Next.js 14? - Intercepting Routes
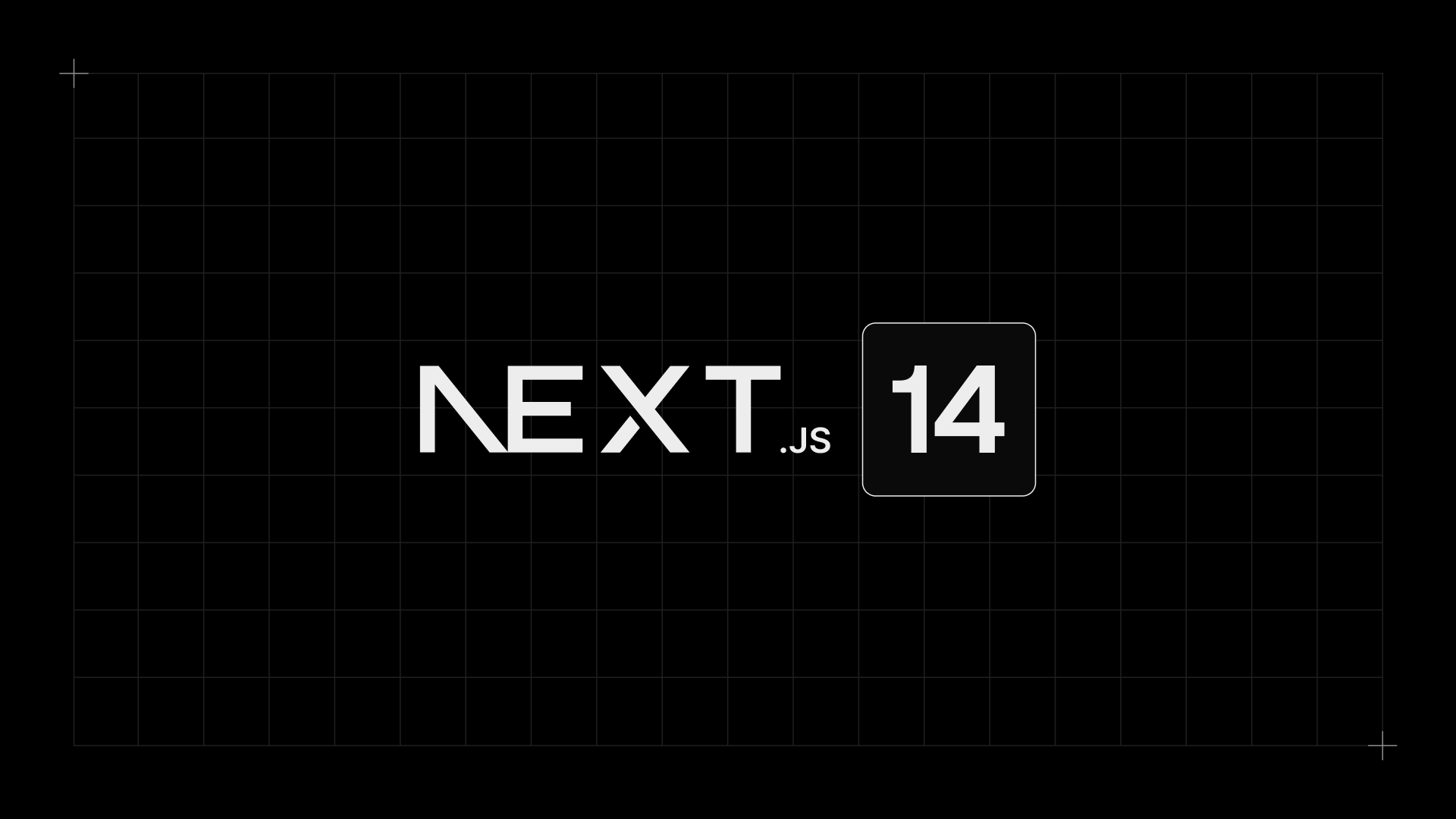
Series: Next.js
- You may not know about Next.js (v13+)
- What's new in Next.js 14? - Intercepting Routes
In version 14, Next.js introduced two advanced routing patterns: Parallel Routes and Intercepting Routes. Within the scope of this article, I will introduce Intercepting Routes. Let's go!
1. What are Intercepting Routes?
Intercepting Routes allows you to intercept or stop default routing behavior to display an alternative view or component when navigating through the UI, while still maintaining the route intended for situations like page reloads. It is often used when you want to display a route while preserving the context of the current page.
Let's take a few examples to make it easier to understand.
Login modal
Suppose you have a navigation bar with a link called /login
, traditionally, clicking on the link will redirect you to the full login page, but with Intercepting Routes, you can displays the login modal while the URL reflects the /login
route, making the link shareable and ensuring the full login page is displayed when reloading the page or accessing directly from the URL.
Message board application
On social networking applications, such as Facebook, when you click on a photo it will usually navigate to a new page dedicated to that image. With Intercepting Routes, clicking on an image opens a modal above the current page, displaying an enlarged image. The URL updates to reflect the selected photo, which is still shareable. Visiting the URL directly or reloading the page will result in a full-page view of the photo.
2. Implement Intercepting Routes in Next.js
First, create two routes /feed
and /feed/photo
, on the feed page, create a Link
tag that redirects to feed/photo
app/feed/page.tsx
: Represents routelocalhost:3000/feed
.app/feed/photo/page.tsx
: Represents routelocalhost:3000/feed/photo
.
The directory structure will be as follows:
import Link from "next/link"
export default function FeedPage() {
return (
<>
<h1>Feed page</h1>
<div>
<Link href="/feed/photo">Photo</Link>
</div>
</>
)
}
export default function PhotoPage() {
return <h1>Photo Page</h1>
}